Hello! I am a Year 2 Computer Engineering student studying in the National University of Singapore (NUS). As someone rather new to the industry, I am always excited to learn and experience new things. I have done both hardware and software projects in my last 2 years in university, and in this document I will be showing you a portfolio of my contributions to my team’s Software Engineering project over this semester.
About The Project
My team and I decided to enhance an existing Command Line Interface address book application to manage camp participants' information, to be used by NUS Freshmen Orientation Camp Project Directors (PDs). This enhanced application allows PDs to view and modify participants' contact details with ease.
In this project, I was in charge of creating the commands that support the addition and modification of groups and houses.
About This Document
This document showcases the features I did, samples of my code, as well as relevant sections I added to the User and Developer Guides.
In this document, the following styles and formatting have been used:
Summary of Contributions
This section shows a summary of my coding, documentation, and other helpful contributions. In this project, I was in charge of creating the logic to support our app’s feature of Group
and House
management.
Major enhancement:
Feature | Description | Justification | Highlights |
---|---|---|---|
Adding, editing and deleting groups and houses |
Allows the user to add, edit and delete |
Being tailored to manage participant details in Freshmen Orientation camps, having groups and houses are essential to organising participants as camp organisers normally do when planning camps. |
These commands are a core component of the project that other features such as |
Minor enhancements:
Feature | Description | Justification |
---|---|---|
Listing participants by houses and groups |
Allows the user to list the participants in a specified house or group. |
Listing the participants in the same group and same house is necessary for the user to filter through data quickly, especially when managing a large camp. It also allows the user to see the current size of a specific group or house. |
Viewing houses and groups |
Allows the user to view the houses and groups they have added so far. |
The exact house or group name must be specified to edit its name, delete it, or add to it. These commands help the user to know which houses and groups have been created so far, and can therefore be added to, modified or deleted. |
Size command |
This command allows the user to view the sizing details of the camp. |
This command allows the user to easily see the number of participants, OGLs, freshmen, groups and houses at one shot, providing a quick way to size the camp. |
Code contributed:
-
Functional code sample: [Add Group Command], [List Group Command]
-
Test code sample: [Edit Group Commmand Test]
-
Check out more of my code on Project Code Dashboard here.
Other contributions:
-
Project Management:
-
In charge of the issue tracker
-
Managed milestones v1.2 to v1.4
-
-
Documentation:
-
Community:
-
Gave consistent feedback to team members to improve their implemented features
-
Gave suggestions for other teams in the same tutorial group to improve their User Guide and Developer Guide
-
Contributions to the User Guide
Our team updated the original AB4 User Guide with instructions for the enhancements that we had added. In this document there are additional icons used, as shown below:
The following is an excerpt from the User Guide showing the additions I made for editing House
and Group
objects:
Editing Houses and Groups
Editing a house : edit_h
Edits a house name.
Format: edit_h OLDHOUSENAME NEWHOUSENAME
Example:
-
edit_h Red green
Edits the house namedRed
toGreen
.
Editing a group : edit_g
Edits a group name.
Format: edit_g OLDGROUPNAME NEWGROUPNAME
Example:
-
edit_g red1 red2
Edits the group namedRED1
toRED2
. All participants in RED1 are now in RED2.
Check out the full User Guide [here].
Contributions to the Developer Guide
Shown below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project.
Group and House Management
FOP Manager supports group and house commands, to reflect the structure of an actual Freshmen Orientation camp. Group and house management refer to how groups and houses are created and modified, as well as viewed, and how they support the addition of participants.
Current Implementation
In FOP Manager, groups can be added, edit and deleted.
Add
-
add_h HOUSENAME
: Adds a new house withHOUSENAME
-
Creates a new house by adding it to
UniqueHouseList
in theVersionedAddressBook
.
-
-
add_g GROUPNAME HOUSENAME
: Adds a new group namedGROUPNAME
into the house-
Adds a new group to a house by adding it to
UniqueGroupList
in theVersionedAddressBook
.
-
The following sequence diagram shows how the Logic
and Model
components interact when the user enters the command add_g r1 red
.
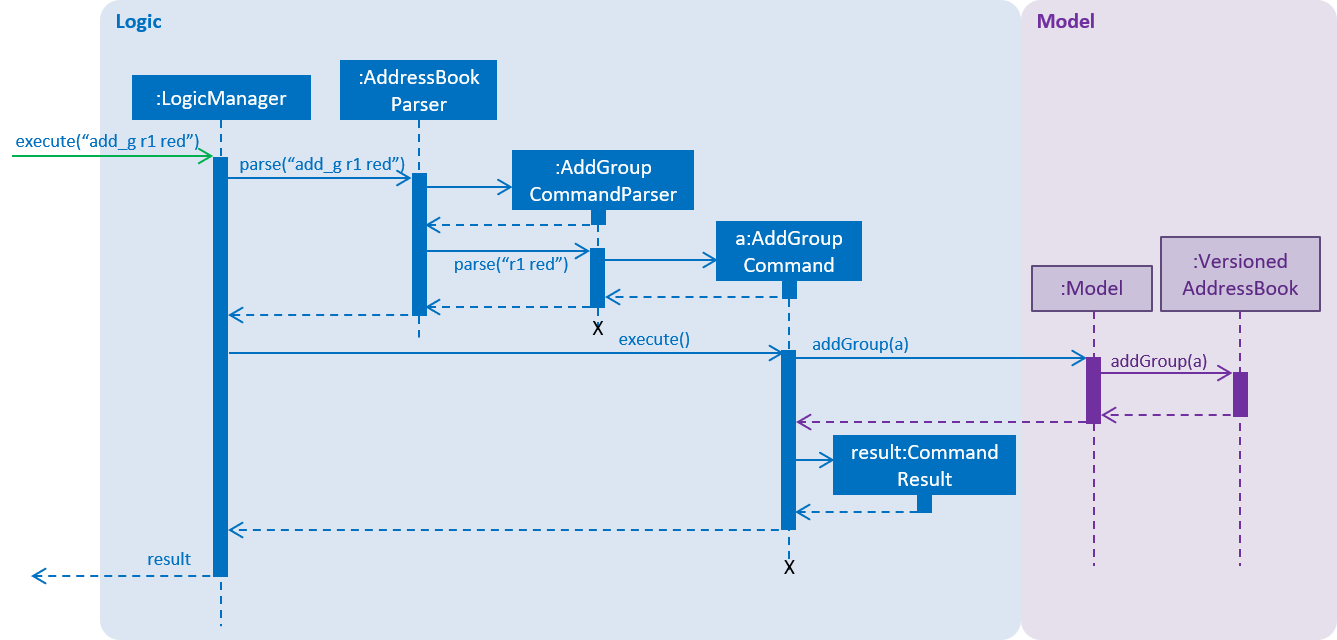
add_g r1 red
-
The new group (R1, Red) is added to
UniqueGroupList
stored withinVersionedAddressBook
when theaddGroup(a)
function is called fromModel
Edit
-
edit_h OLDHOUSENAME NEWHOUSENAME
: Edits the name of an existing house-
Edits the name of a house in
UniqueHouseList
, as well as changes the house name of all groups within the house inUniqueGroupList
.
-
-
edit_g OLDGROUPNAME NEWGROUPNAME
: Edits the name of an existing group-
edit_g
edits the name of a group inUniqueGroupList
, as well as changes the group name of all participants with that group name inUniqueParticipantList
.
-
The following sequence diagram shows how the Logic
and Model
components interact when the user enters the command edit_g r1 r2
.
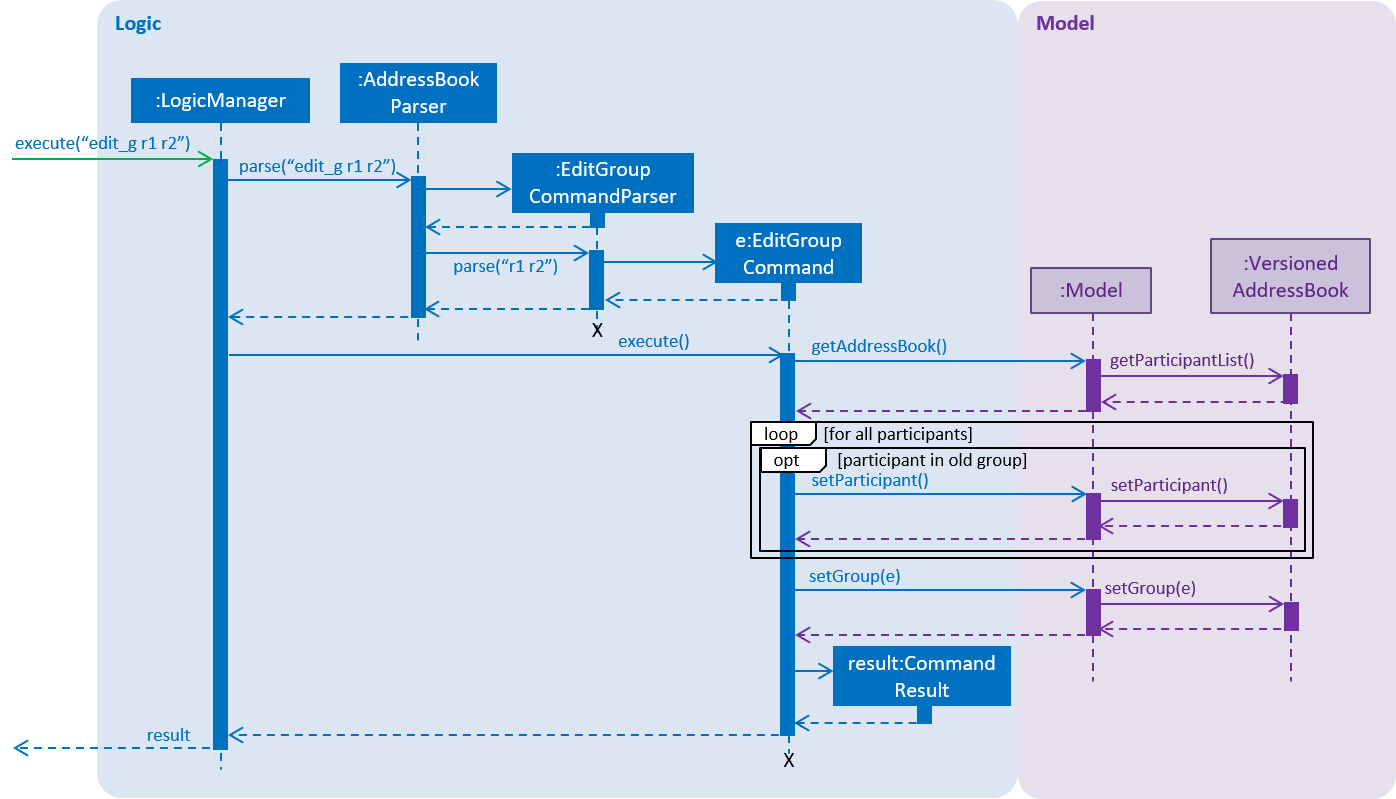
edit_g r1 r2
-
The edit group command updates the group of all participants within the old group by looping through
UniqueParticipantList
fromVersionedAddressBook
to check if their group matches the old group name. -
This command also changes the name of the group within
UniqueGroupList
stored withinVersionedAddressBook
.
Delete
-
delete_h HOUSENAME
: Deletes the group namedHOUSENAME
-
Deleting of house objects require there to be no groups within that house.
-
-
delete_g GROUPNAME
: Deletes the group namedGROUPNAME
-
Deleting of group objects require there to be no participants within that group.
-
Groups and houses can also be viewed in different manners:
-
Viewing houses and groups
-
view_h
andview_g
simply displays the houses and groups stored inUniqueHouseList
andUniqueGroupList
respectively.
-
-
Listing participants in a particular group or house
-
list_g
andlist_h
use similar logic tolist_o
andlist_f
, implemented by using an entered group name as a predicate that searches through all theGroup
fields of participants, and updates thefilteredParticipant
list with participants with the matching group name or house name respectively.
-
list_g empty lists all participants without a group by searching for participants with an empty group name.
|
Design Considerations
Aspect: Storage of group and house lists
Alternatives | Pros | Cons |
---|---|---|
Storing groups and houses within a single list of houses that contain differing number of groups. |
Easy management of data |
Requires looping through all houses to find a single group and to ensure group names are unique |
Storing groups and houses in 2 separate lists (current choice). |
House objects are not affected by the operations done to the groups stored within it |
More computationally expensive to identify the groups within a single house |
We decided to follow the second alternative as it allows us to identify a specific group faster, a function that will be more commonly used in FOP Manager, since it is called when adding and editing a person and/or a group name.
Aspect: Deleting a group object requirements
Alternatives | Pros | Cons |
---|---|---|
No requirements when groups are deleted. |
User can delete a group much more easily |
Participants in the deleted group will belong to no group |
Requires a group to contain no participants before it can be deleted (current choice). |
User can be sure to not delete a group with participants in it accidentally |
Participants must be manually removed from the group before being deleted |
Camp organizers rarely intend to remove a filled group. Requiring a group to be empty before being deleted acts as a fail-safe to ensure that filled groups are not accidentally removed, resulting in participants having to be manually added back to the group.
Aspect: Duplicate group names under different houses
Alternatives | Pros | Cons |
---|---|---|
Duplicate group names are not allowed (current choice). |
Groups can be searched for by name without specifying its house |
Simple names such as 1 and 2 cannot be used for different houses at initial planning stage |
Duplicate group names under different houses are allowed. |
User can easily name groups without too much thought |
User must always specify house name when searching for a specific group |
We chose to not allow duplicate groups, as it makes the app more user-friendly. Moreover, this will model real life situations as camp groups normally have unique group names.
Check out the full Developer Guide [here].