INTRODUCTION:
Hello! My name is Wei Zhang but you may call me Clyde for short. I’m currently a second year student in NUS School
of Computing, pursuing a Bachelor of Computing in Information Security.
At the time when this document was created (over a period of Jan 2019 to April 2019),
I took CS2113T (Software Engineering & Object-Oriented Programming) and CCS2101 Effective Communication
for Computing Professionals, which requires me to develop a desktop application and write documentations for it
as part of the curriculum.
I held various roles in the development of this project which includes designing the application architecture,
developing core features, code integration and testing.
Aside from this project, I’m also a self-taught Cyber Security Practitioner where my main interests are
Penetration Testing and Digital Forensics.
ABOUT:
The purpose of this document is to showcase the contribution of my work towards this
project as part of the learning in this module.
The outline of this document will be listed as follows, mainly describing the work I have done in both technical
and non-technical aspect:
-
Summary of Contributions: Describes mainly the technical aspect of work I have contributing in the development of the project. It also includes any other possible contributions I have done that may not be technical.
-
Contributions to User Guide: Showcases my ability in writing a guide for user of the application that was developed for.
-
Contributions to Developer Guide: Showcases my ability in writing a guide for developers who may continue to work on the development and maintenance of the application in the future.
Overview
FOP Manager is a desktop application developed for NUS undergraduate students who are Project Directors of Freshmen Orientation Programs to manage both participants and committee members of the program. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC. The development of this application is to teach Principles of Software Engineering, which is part of the context of CS2113T.
Summary of contributions
This segment showcase my contribution to both the development of the application and
the documentation of the project.
-
Major enhancement:
Description |
Justification |
Highlights |
|
Randomize Command |
A command that allows the Project Director to automatically assign all participants to created groups. |
This allows the Project Director to automatically assigns all participants to created group at a random distribution without doing it manually. |
The challenge here is to come out with a suitable algorithm which ensure even distribution and no possible loop holes (e.g. all OGLs in 1 group). |
Redesign Model and Storage component |
Redesigning the way the application stores and manages data |
This enables the storing and managing of house and groups on top of the current participants. |
The challenge here is figuring out how is the whole application designed as this modification requires knowing the code base very well. |
-
Minor enhancement:
Description |
Justification |
Highlights |
|
Input box is clear after every command execution |
Simulate a Terminal/CMD (i.e. Command line Interface in Windows/Linux) interface which clears the input segment after every entering of commands (i.e. text is cleared when the user hits the 'enter' button). |
This improves the user friendliness of using the command line as the target users prefers using CLI (Command line Interface) over GUI (Graphical User Interface). |
The challenge here is to figure out the code for the UI component and ensuring which part of it is to be placed. This include ensuring the fix works as well. |
-
Code contributed:
-
Other contributions:
-
Project management:
-
Reviewing most of the codes before officially merging and integrating
-
Setting up of the main project repository (GitHub)
-
Setting up continuous integration technology (TravisCI)
-
Setting up of code contribution analysis tool (RepoSense)
-
Managed release for v1.3 and v1.4 on GitHub
-
-
Documentation:
-
Contributions to the User Guide
This segment showcases my ability to write documents for end users based on the work that I have contributed. |
Randomizing groupings : randomize
Randomize group allocation of all registered participants.
Format: randomize
Examples:
-
randomize
Successful Output:
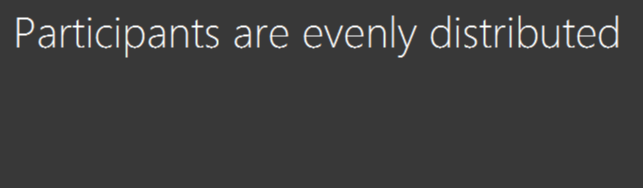
-
randomize
.
Error Output:

Contributions to the Developer Guide
This segment showcases my ability to write technical documents explaining the underlying technicalities for developers based on the work that I have contributed. |
Randomized Group Allocation
FOP Manager has a randomize
command, which allows the Project Director to automatically assign all participants to a group.
Following is the activity diagram when the command is executed:
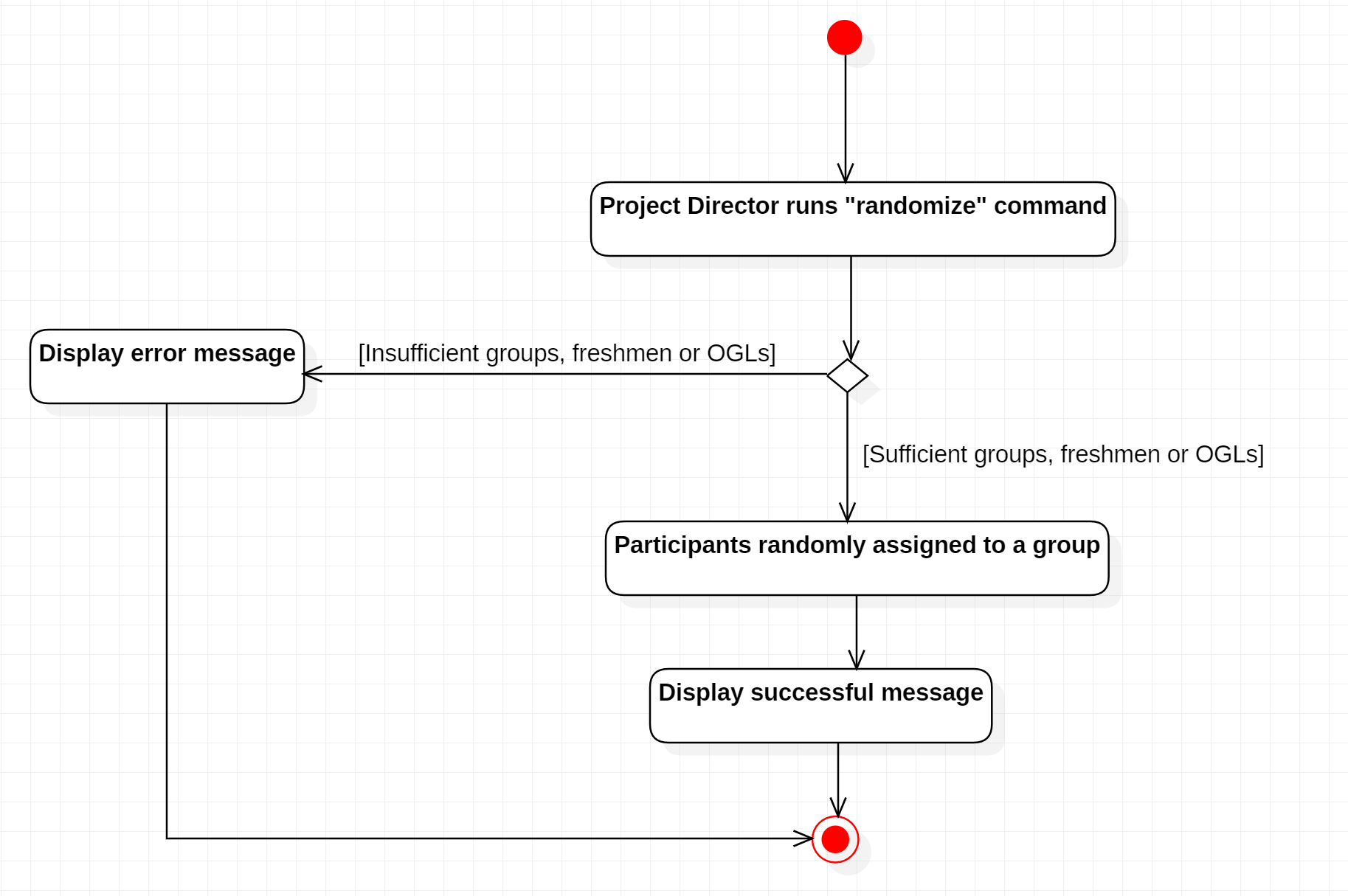
Current Implementation
The randomize
is achieved by coding a sequence of steps to achieve the allocation of groups to participants:
-
Creating list holding specific type of participants (i.e. all OGLs will be stored in a list while freshmen will be stored in another)
-
Shuffles all of the previously created list
-
Assigns all of the participants to a group in order of freshmen, OGLs
Following is the sequence diagram of the flow of the program when the command is executed:
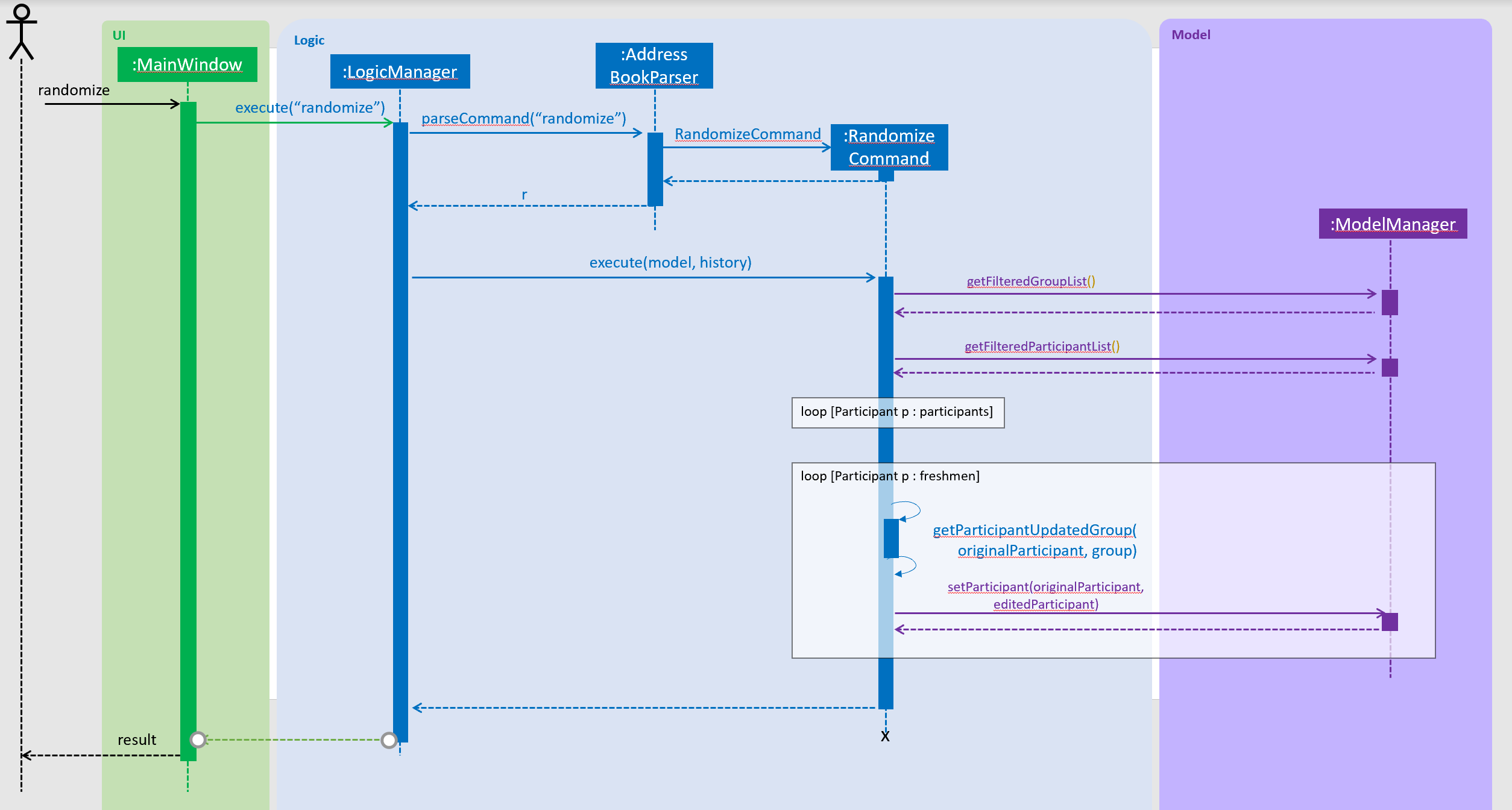
The above Sequence Diagram only shows the updating of group details for freshmen (for clarity purposes, refer to the codes for more details). The algorithm is applied also to OGLs within the RandomizeCommand class. |
Algorithm
This segment will show relevant diagrams explaining the algorithm for the shuffling of assignment and cases where the randomization will not happen. The following diagram shows the annotations and symbols that will be used:
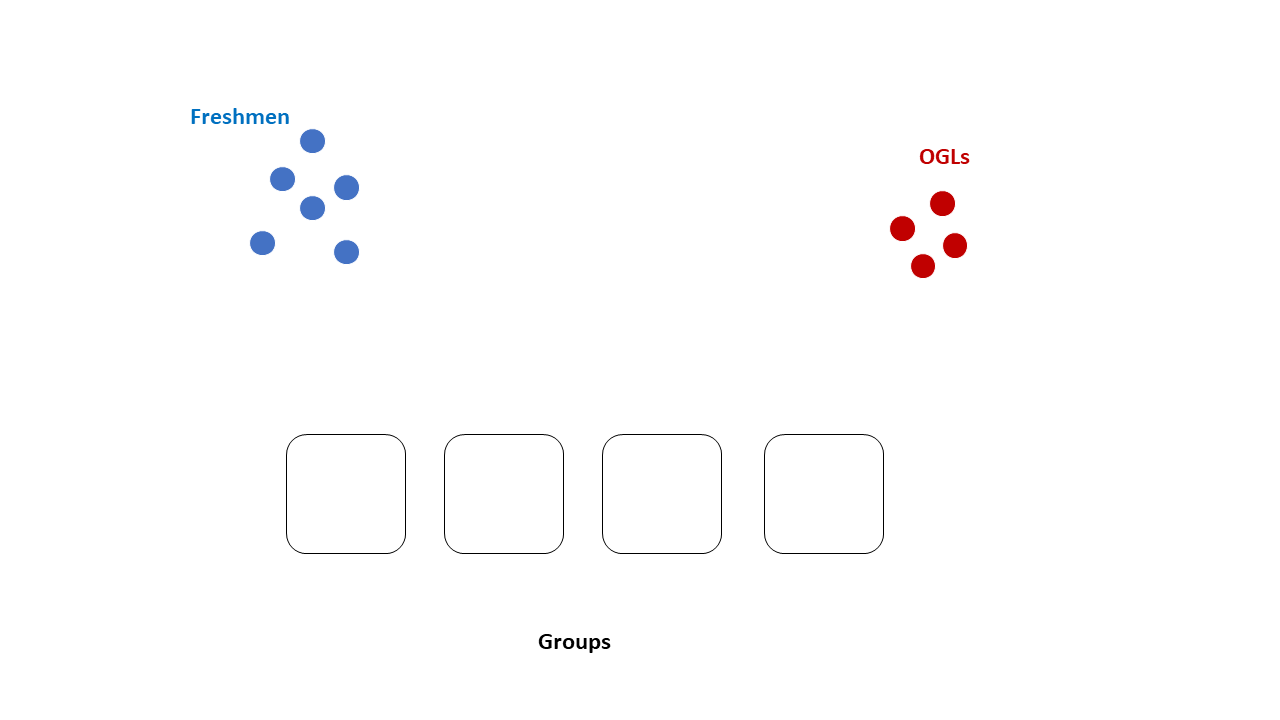
The following diagram shows an example where the Project Director has added sufficient OGLs and freshmen to the system, and created sufficient groups:
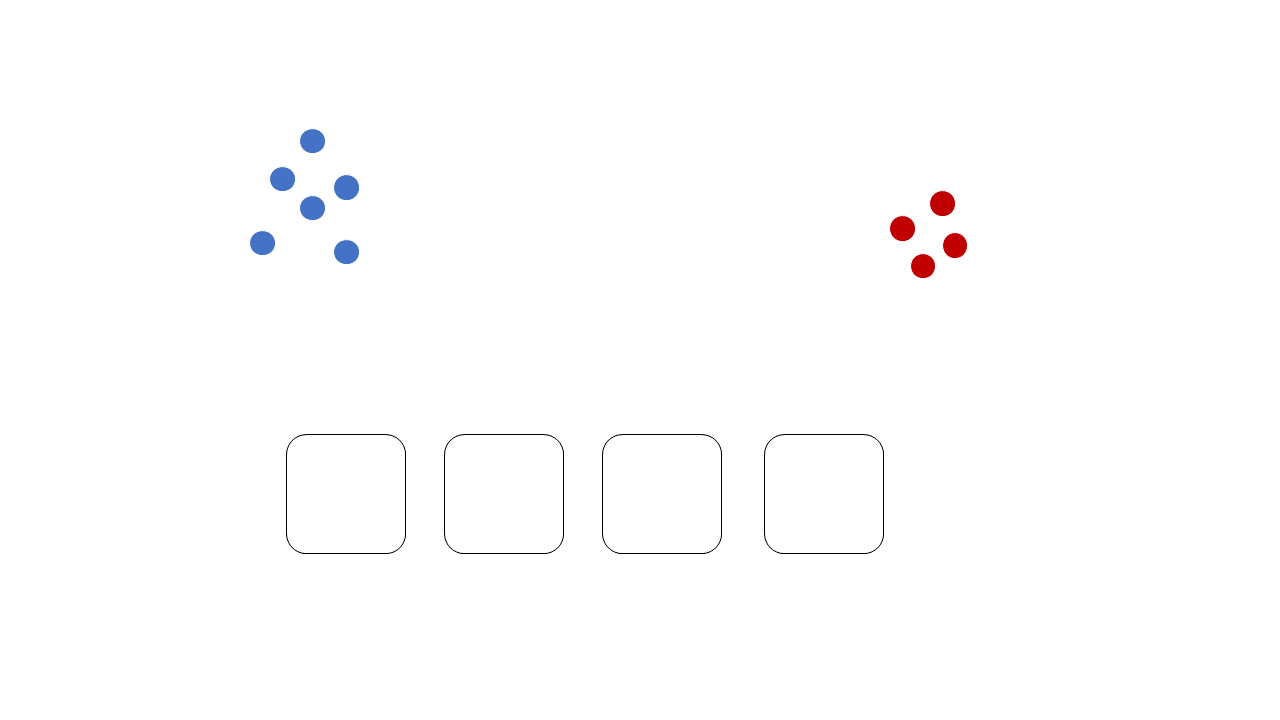
Upon executing the randomize
command, the system will first assign the freshmen to the groups first:
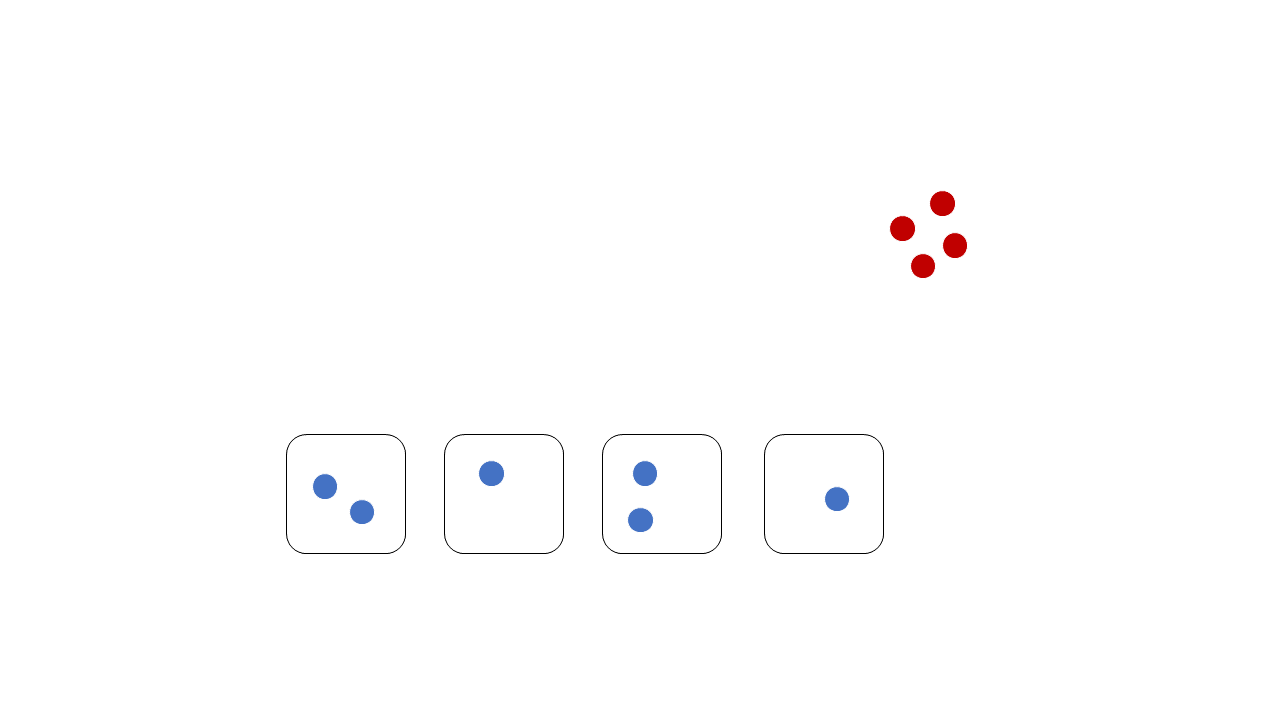
Followed by assigning the OGLs to the group:
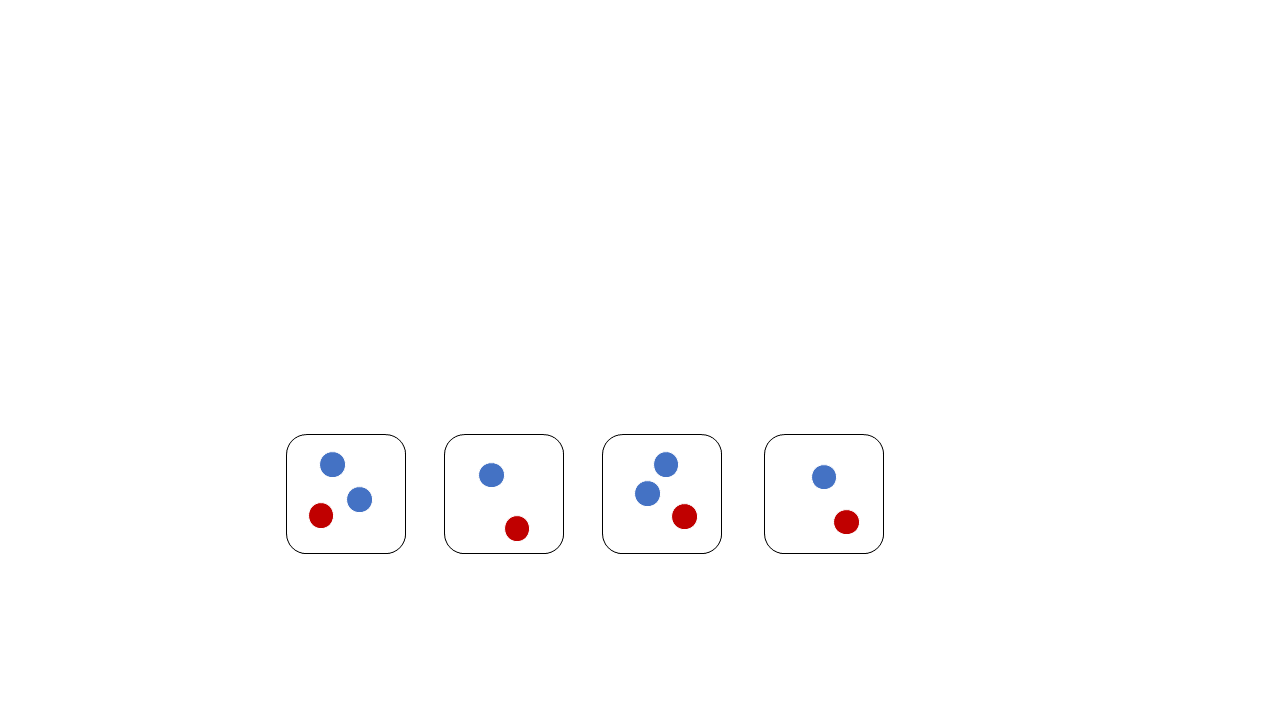
The rationale for such assignment is to ensure that the every group has an OGL. |
Following diagrams shows the cases where the randomization will not happen and will throws an error message backs to the user.
Insufficient participants:
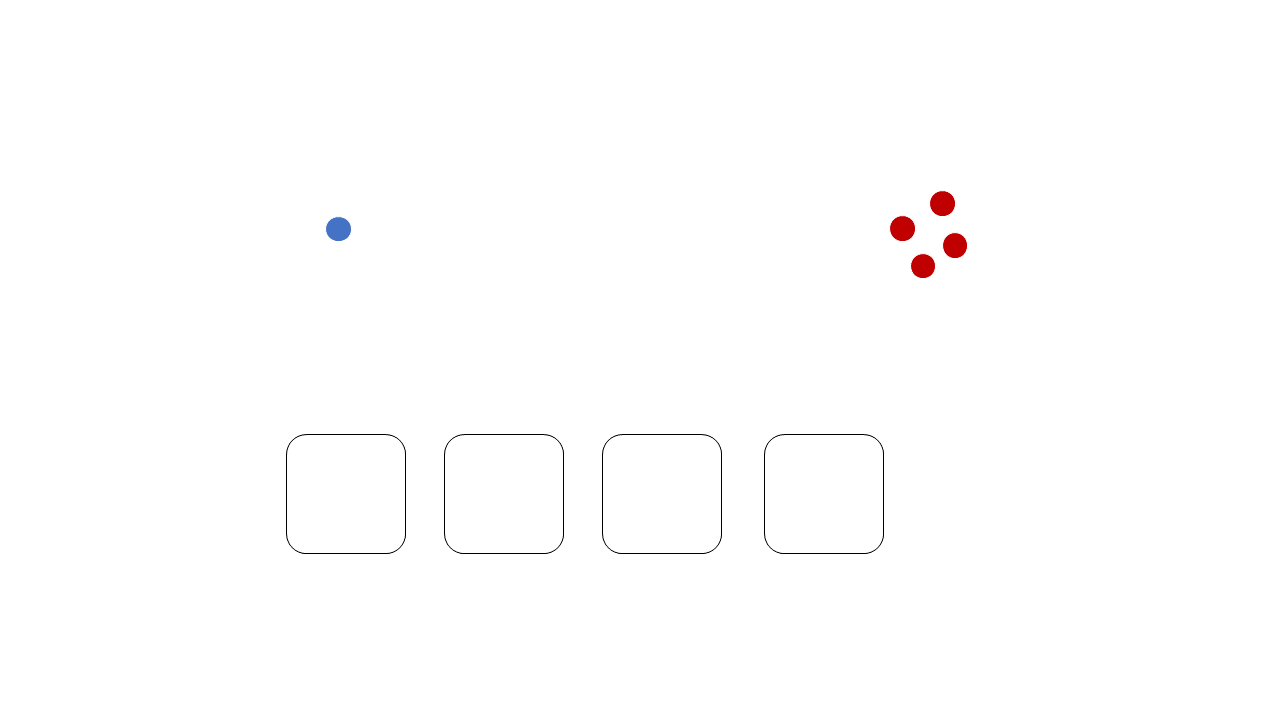
Insufficient OGLs to cover all the groups:
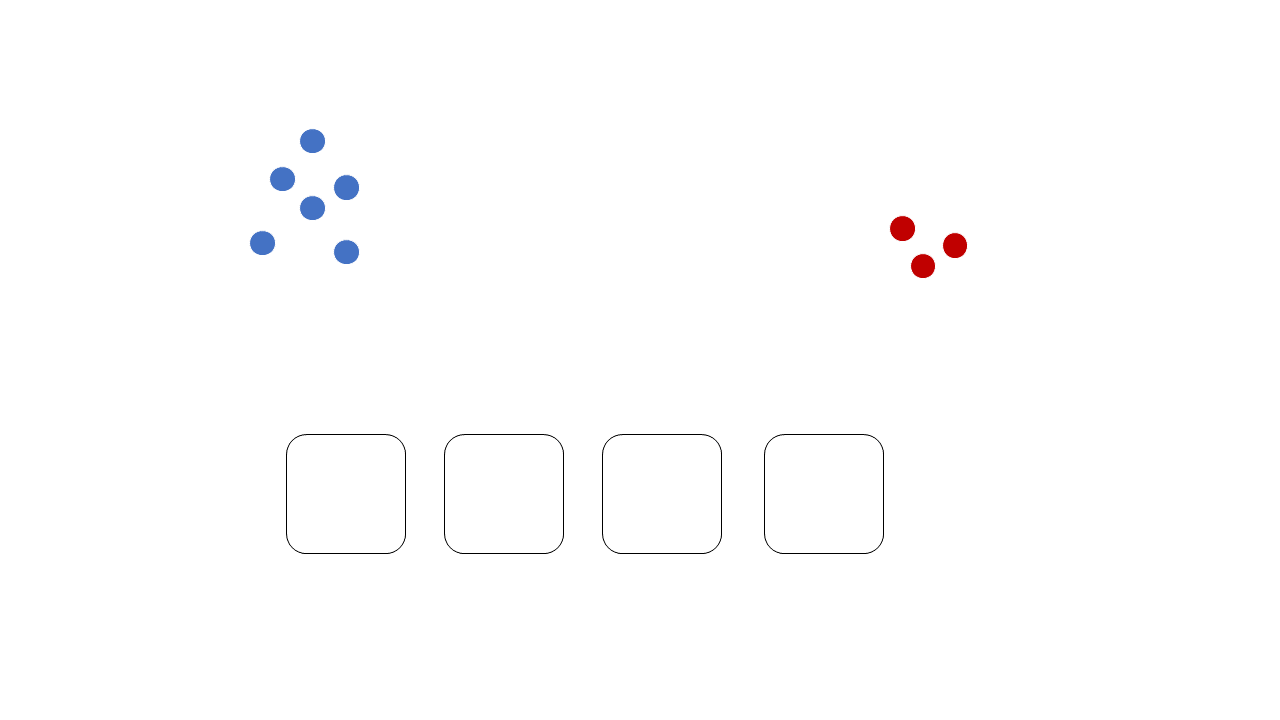
Insufficient groups:
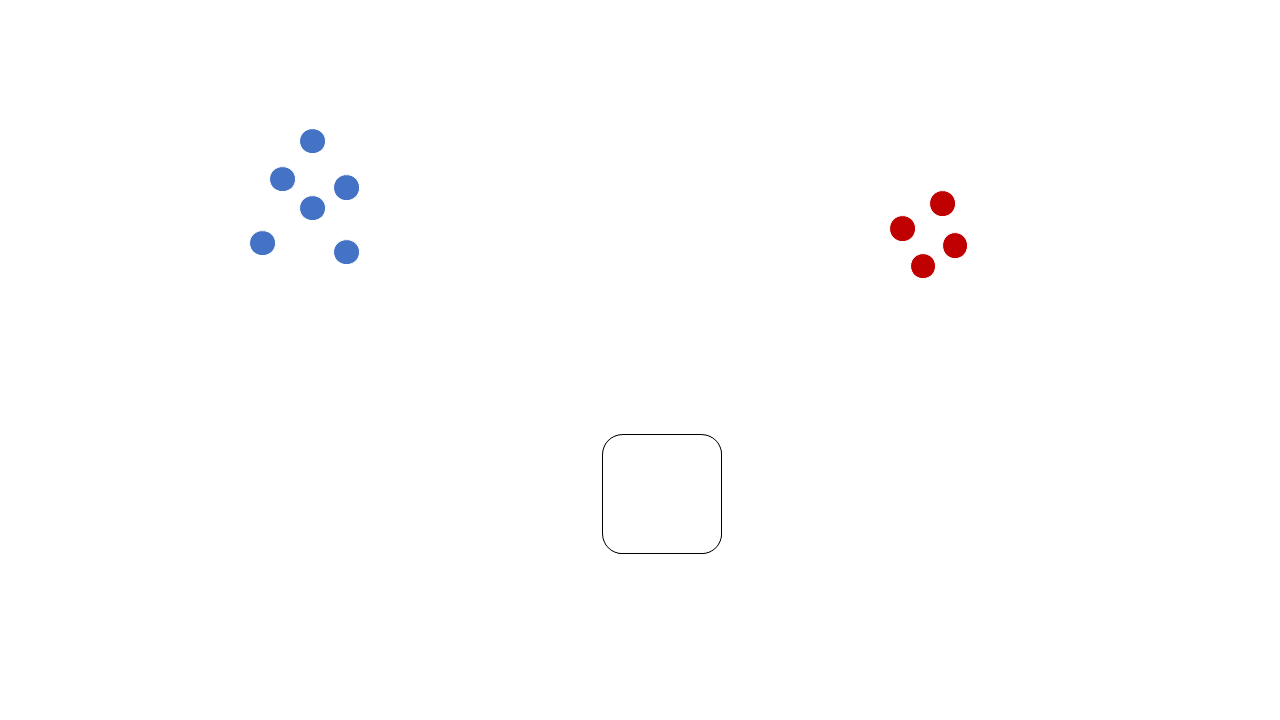
Engineers are free to modify the algorithm for the random distribution but are advised to consider the corner cases similarly to the ones mentioned above. |
Design Considerations
Aspect: Updating group attribute of participants
Alternatives | Pros | Cons |
---|---|---|
Programmatically simulate the calling of the |
Changing of interface with the Model component only requires changing the EditCommand and Parser |
Tightly coupled with EditCommand and EditCommandParser |
Calls the function that edit the details of the participants by interacting with the Model component (current choice) |
Implementation is obvious, follow guidelines of the system architecture and cleaner code base |
Coupled with ModelManager |
Rationale: It is to follow the design architecture of the system, allowing it to be consistent with
the rest of the codes and cleaner code base. It may seems intuitive for some engineers
to programmatically simulate the edit
command,
but it might causes unknown complexity within the system if there is a change in the dependent classes.
Aspect: Shuffling of participants
Alternatives | Pros | Cons |
---|---|---|
Creating a list fo freshmen and OGLs, then shuffles the list separately (current choice) |
Ensures all groups will have at least an OGL and balanced distribution amongst the group |
Requires more memory space |
Shuffling the list of participant |
Saves memory space |
Risk having uneven distribution (e.g. all OGLs in a group) |
Rationale: The idea of separating freshmen and OGLs is to ensure that every group will have at least 1 type of participant. By shuffling the list of participant containing both freshmen and OGLs, we risk running into a situation where there are groups with no OGLs, which is something that a Project Director does not want. The current choice ensures that all of the OGLs will be distributed evenly into groups similarly to how the freshmen are distributed.
Before the randomization happens, the application ensures that there are sufficient OGLs to cover all of the created groups (i.e. at least 1 OGL per group). Refer to the codes for more details. |